In this tutorial, we will show you the step-by-step procedure to try out object-relational mapping by first, define the data model in ERD with sample data, then generate class diagram from ERD, generate database and hibernate code and finally use the generated hibernate code to insert data to database and retrieve data from database. As the focus of this tutorial is how you can work with hibernate together with Eclipse and Visual Paradigm, a minimal example will be used. Visual Paradigm, Eclipse 8.4.1 Luna and Microsoft SQL Server will be used in this tutorial.
Getting started
We assume you have the SQL Server, Eclipse and Visual Paradigm being installed. Now let's get started. We first create a database in our SQL Server.
Setup your database
To setup a database in SQL Server:
- Login your SQL Server via Microsoft SQL Server Management Studio.
- Right-click on the Databases node in Object Explorer and select New Database... from the popup menu.
- Enter the name of the database. In this tutorial, we name the database as ToyStore.
- Click OK to create the database.
Creating Java project in Eclipse
- Start the Eclipse IDE.
- Click the New button in Application Toolbar and select Java Project to open the New Java Project window.
- In the New Java Project window, enter Toy Store in Project name field.
- Click Finish to create the project.
Starting Visual Paradigm in Eclipse
Now, we have to start up Visual Paradigm in Eclipse.
- Right-click on your Java project and select Open Visual Paradigm from the popup menu.
- You may be prompted to specify the location of your Visual Paradigm project. In this case, simply select Create in default path and click OK to proceed.
- When you are prompted to switch to the Modeling perspective, choose Yes.
Configure database for your Eclipse project
Before the modeling start, we have to specify the database configuration for our project first.
- Select Modeling > ORM > Database Configuration... from the main menu
- Select MS SQL Server from the database list.
- Select 2008 or higher in the Version field.
- Leave the Driver field unchanged (selecting jDTS Driver), then press the green down arrow button to download the required driver. You may need to specify your proxy server for accessing Internet and for downloading the driver.
- Next, fill in the hostname, port number, the Database name as well as your User name and Password to access for the database.
- Click Test Connection to make sure the connection setting we defined is correct.
Now, everything is ready and we can start creating our data model.
Create data model with ERD
We can start create data model for our project.
- Right-click on Entity Relationship Diagram in Diagram Navigator and select New Entity Relationship Diagram from the popup menu.
- Select Entity in the diagram toolbar and click on the diagram to create an entity. Name it Category.
- Right-click on the entity and select New Column.
- Enter +ID : int to create a primary key with ID as name and int as type.
- Press Enter to confirm and create one more column. Enter name : varchar(255) as column name. Press Enter again to confirm and press Esc to cancel editing.
- Move the mouse pointer over the Category entity. Press and drag out the Resource Catalog icon at top right.
- Release the mouse button and select One-to-Many Relationship -> Entity from Resource Catalog.
- Name the entity Product.
- Create three columns to Product, +ID : int as primary key, name : varchar(100) for the name of the products, and stockQTY : int for the availability of the products.
Creating sample data
We can fill in some sample data for our data model in ERD. This will allow those sample data to be generated to database along with your database schema, which can be very useful in system testing.
- Right-click on the blank area of the ERD and select Show Table Record Editor or View Editor from the popup menu.
- Select the entity Category in diagram and enter two sample categories in the editor - Car and TV Game.
- Now, select the entity Product and create two sample products - the Diecast Sport Car with quantity 100, which is under the Car category; and the All Sport Package with quantity 450, which is under the TV Game category. You can click the ... button in the foreign column (i.e. Category) to open the selected category which you have defined instead of manually entering its value.
Generate class model from ERD
We have finished designing the database with ERD. Now, we can generate a class model from it. To generate the class model out of your ERD:
- Select Modeling > ORM > Synchronize to Class Diagram from the main menu.
- Click OK in the Synchronize from Entity Relationship Diagram to Class Diagram window.
- Click OK again in the Synchronize to Class Diagram window.
- The class diagram is generated. Click the package header <default package> and enter toystore as the package our class model. Without specifying a package, all the classes will be placed to the project root, which may make it hard to manage.
The data models are ready and we can proceed to generating Hibernate source code as well as the database.
Generate code and database
To generate Hibernate source code and the database:
- Select Modeling > ORM > Generate Code... from the main menu.
- Make sure we have selected Code and Database in the Generate field.
- In the Code tab, select JPA as the persistence Framework.
- Select DAO as the Persistent API.
- Switch to the Database tab and select Export to database to have the database schema directly generated to database.
- In the Generate Sample Data field, select Yes (With Auto Generated PK) for having sample data automatically inserted into database.
- Click OK to proceed.
After that, the tables with their sample data will be generated directly to the database. You can view them in the SQL Server Management Studio.
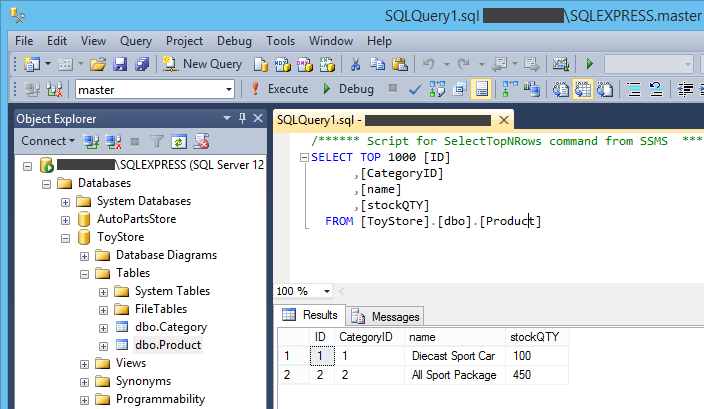
Programming with generated Hibernate code
Here comes the core part of this tutorial - to use the generated Hibernate code.
Inserting records to database
- Open the ormsamples.CreateToyStoreData.java.
- Let's comment out the code that inserts sample data into the database, and write our own code.
-
The sample code already has the basic template for objects creation. Let's modify them to insert our own data. Let's define the name for the instance ltoystoreCategory as RC Model by using the setter method.
PersistentTransaction t = toystore.ToyStorePersistentManager.instance() .getSession().beginTransaction(); try { toystore.Category ltoystoreCategory = toystore.CategoryDAO.createCategory(); ltoystoreCategory.setName("RC Model"); ...
-
For the ltoystoreProduct, specify its name as 1:24 RC Car with quantity 300.
... toystore.Product ltoystoreProduct = toystore.ProductDAO.createProduct(); ltoystoreProduct.setName("1:24 RC Car"); ltoystoreProduct.setStockQTY(300); ...
-
Next, we are going to associate the ltoystoreCategory with ltoystoreProduct. Since one category contains many products (remember that we defined it as one-to-many relationship?), we can associate them by using the collection in the generated code. The name of the collection is generated based on the role name in the association, in this case, it is the products variable in Category class.
... ltoystoreCategory.product.add(ltoystoreProduct); ...
Your code should look like the following. - Now, let's try out the program. Right-click on the blank area of the code editor and select Run As > Java Application from the popup menu.
- Open SQL Server Management Studio again and you will find that the RC Model category and the 1:24 RC Car product being inserted into database.
Retrieving records from database
Let's retrieve data using the Hibernate code.
- Open the ListToyStoreData.java.
- By default, the sample code will perform query on object type one by one. But since our Category class and Product class are related to each other, we can make use of the association to retrieve them from database instead of query them in individual request. Let's comment on the section of querying the products in the sample.
-
Edit the sample code on querying the category and append the code for printing its name.
... int length = Math.min(ltoystoreCategory.length, ROW_COUNT); for (int i = 0; i < length; i++) { System.out.println(toystoreCategorys[i].getName()); System.out.println("Containing Products:"); ...
-
Next, we retrieve the product collection from category and then convert it to an array of Product objects.
... Product[] products = toystoreCategorys[i].product.toArray(); ...
-
Now, loop through the array to printout the information of the Product which is associated with the Category.
... for (int j = 0; j < products.length; j++) { System.out.println(products[j].getName() + ", QTY: " + products[j].getStockQTY()); } ...
- Right-click on the blank area of the code editor and select Run As > Java Application from the popup menu to try out the sample.
You can see the details of the Category as well as its containing Products listed out in the Output window.
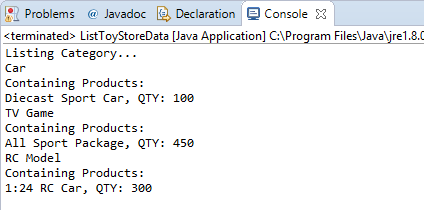